Sometimes it happens to store data in the device storage and not in a db. In this post we will see how to do it in Flutter with Shared Preferences.
The package SharedPreferences
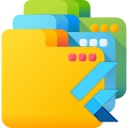
In Flutter we use SharedPreferences to store the App preferences in the local memory of the device.
Link to the repository: https://pub.dev/packages/shared_preferences
How to install the package?
First thing to do is to add the packet between the plugins in pubspec.yaml file:
dependencies:
flutter:
sdk: flutter
shared_preferences: "latest-version"
At the top of dart file where we want to use the SharedPreferences we have to import the library:
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
Types of data we can store
With this package data are store as a couple of (<key>,<value>) and the supported types of data are int, double, bool, String e StringList.
The operations supported by Shared Preferences
This packet supports three types of operations:
Read data from the local storage
The method to read a SharedPreferences stored changes with respect to the type of data that we want to read. It can be getInt(), getBool(), getString() or getStringList().
Let’s see an example with getStringList():
//we define the variable to access SharedPreferences
final prefs = await SharedPreferences.getInstance();
//We retrieve preferences and we store in a string list
List prefList = prefs.getStringList("prefListInStorage");
Obviously if we have never stored any preferences in the local memory this list is null.
Store data in the local storage
The method to store a SharedPreferences changes with respect to the type of data that we want to read. It can be getInt(), getBool(), getString() or getStringList().
Let’s see an example with getStringList():
//we define the variable to access SharedPreferences
final prefs = await SharedPreferences.getInstance();
//We retrieve preferences and we store in a string list
List prefList = prefs.getStringList("prefListInStorage");
//different operations on the list
//we store the new value of the SharedPreferences in the local memory
prefs.setStringList("prefListInStorage",prefList)
Every time that a variation on data that belongs to a SharedPreferences is done we must store the new value in the local memory.
Remove data from the local storage
The method to remove a SharedPreferences is unique and it is called remove().
Let’s see an example:
//we define the variable to access SharedPreferences
final prefs = await SharedPreferences.getInstance();
//We retrieve preferences and we store in a string list
List prefList = prefs.getStringList("prefListInStorage");
//different operations on the list
//we remove the list from SharedPreferences
prefs.remove("prefListInStorage");
A practical example: App options
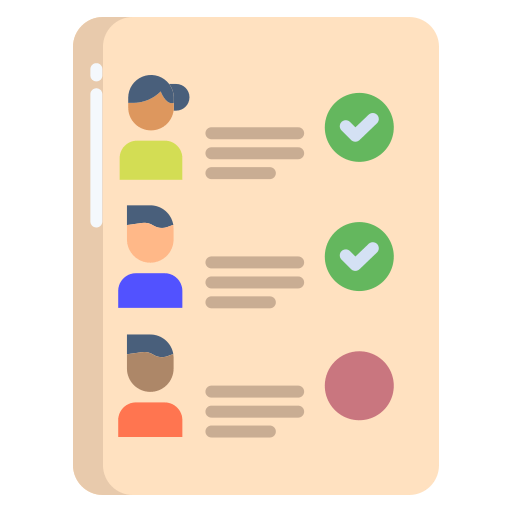
Suppose to have an App with a list of options that a user can choose.
These options can be stored in the local memory of the device with a SharedPreferences and they will be updated every time a user changes them.